Introduction
You probably have heard about ‘APIs’ a million times by now, But what are they? Why should they matter? and more importantly, how can they help you?
In this article, we will cover the following:
- What an API is.
- How to make API integrations
- How to troubleshoot an error.
What is an API?
An application programming interface or API (as it is fondly called) is an interface that allows you to communicate with other applications and data sets. You can think of APIs as a doorway to another application or data.
When a system wants to share its application with an external system, it would expose API endpoints for that application. This way, other developers can connect their applications to the ‘shared’ application.
APIs power a lot of popular websites, web and mobile apps. They are really helpful in extending functionalities into these apps. Some APIs help you collect payments, get locations on maps and lots more!
How do APIs work?
APIs are a bunch of rules that allow applications to communicate with each other. To understand better why we need these rules, let’s take a look at the components involved in this communication:
- A User – This is the person who triggers the communication.
- A Client – This is an application through which the user sends the communication
- A Server – This is usually the computer that houses the application you want to connect with.
A developer would first build the server to hold the application. This server would process and hold application data. Once this is done, the developer would publish documentation that would guide on how other applications can get connected to the server and get its application data.
This API communication is usually in two phases. The first part is called a request. This is from the client to the server. After a request is made, the server then sends back the application data. The communication from the server back to the client is called a response.
What makes an API?
Now that we know what an API is, let’s take a deeper dive into the different parts that make up the API.
Endpoints
An Endpoint is a URL that serves as entry points to an API. You can think of it as a specific URL for a function on a server. For example, to get your account balance with Flutterwave, You need to interact with that part of our application. It is exposed via the URL, https://api.flutterwave.com/v3/balances
HTTP Verbs
Just like grammatical verbs, HTTP verbs are actions that an API can make. Today, there are lots of verbs you can use, but we would be focusing on the popular ones.
- Get – When the GET method is used with an API request, It allows the user to fetch information from the server. This method is especially useful for querying and downloading information from the application server.
- Post – The POST method is the literal opposite of the GET method. This method allows sending information to the server. The method is used mainly for creating a new resource in the server e.g. creating a new payment.
- Put – This is only used when the user intends to update existing information (or information sent previously to the server).
- Delete – This is only used to permanently remove information from the server. This should be used after careful consideration.
Body Parameters
When sending a POST request, you would need to send some information in the body of this request. Any information that you do not pass in the API URL or header is by default sent in the body of the request.
The body of the request is usually in JSON (JavaScript Object Notation – a dictionary, key-value pair) or XML format.
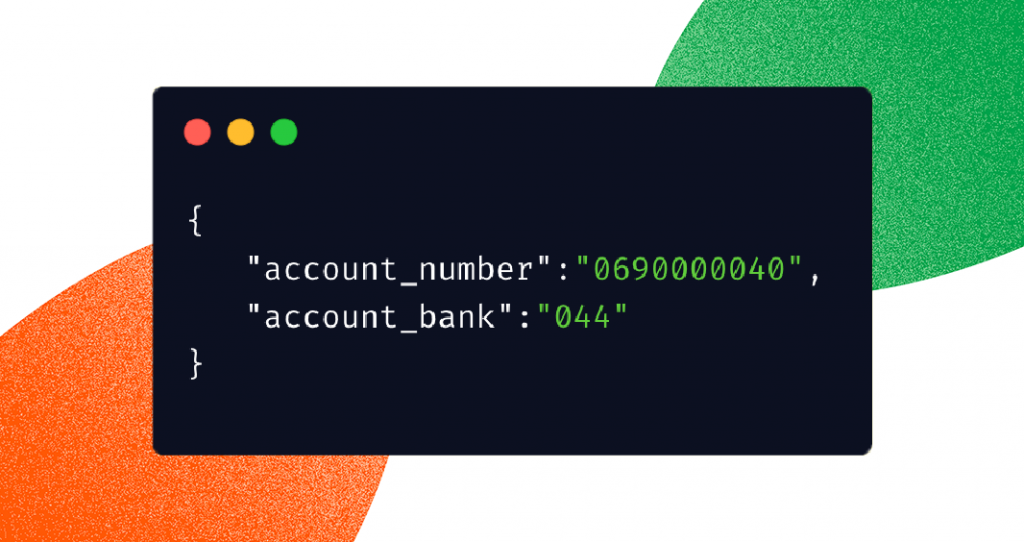
Query Parameters
Query parameters are parameters found only in the endpoint URL. They are used as filters to fine-tune the data being returned from the server. Query parameters are separated from the main URL by a question mark.
Path Parameters
These parameters behave just like variables in the endpoint URL. They are useful in carrying out dynamic actions on multiple items. For instance, the verify endpoint (https://api.flutterwave.com/v3/transactions/:id/verify) contains the path parameter – ID. This allows the user to pass the IDs for different transactions to this endpoint.
Working with APIs
Authentication
Most APIs require you to sign up to get a key (this could sometimes be a combination of different keys) to access the server. This is important for several reasons, some include:
- To protect the data.
- To eliminate the abuse of the data stored or processed on the server.
The API keys help the server identify which user is making a request. This makes account profiling and access to different levels of APIs possible.
When making an API call, always refer to the developer documentation. This would explain how you can get your Keys and authenticate the API requests.
Making an API request
In this section, we’d quickly cover the essentials of making an API request.
Pre-requisites
To get started with your API calls, Here is a helpful checklist to get started.
- Sign up and get your API Keys.
- Read the Developer docs (Just the API reference for the feature you need is sufficient).
- Decide if to make the call via an API client or from your code.
Using an API Client
This is an easy way to start exploring APIs. API clients are tools that can help you in making requests. Popular clients include browsers(e.g. Google Chrome), terminals and custom clients (e.g. Postman, Insomnia).
For this demonstration, we would make use of the Flutterwave transfer API
- Select the HTTP verb for the API call.
- Enter the API endpoint into the URL bar.
- Switch to the Header tab and add the authorization header. For this demonstration, add Authorization as the key and Bearer “your secret key” for the value.
- Next, head back to the body tab and select JSON as the format for your request.
- Add the body payload.
- Click on the Send button to initiate the API request.
You would receive a response to the API request after following the steps. The response would contain information from the server regarding the payout you just made.
Using Code
Depending on the language being used in your code, there are lots of libraries that can help you with your API requests. We have listed a few good ones below. Be sure to check out their documentation for more details on their use.
- JavaScript – Axios, node-fetch
- PHP – Guzzle, Requests, Unirest PHP
- Ruby – Rest-Client, http.rb
- Python – Requests (not to be confused with PHP’s request library), http
Troubleshooting API Errors
API Errors can occur on either side of the communication i.e. on the client-side and server-side. In this section, we would be discussing how to debug common errors you may encounter when making your calls.
Here are some handy tips for resolving API errors on your client (Browser or Postman):
- Check your internet connection.
- Check your firewall rules and security settings.
- Consult the API documentation to confirm that the endpoint URL and API body have no errors.
- Checking the Network tab.
- Review the status code returned in the response.
Next steps…
Well done for making it to the end. With these basics, you can start interacting with APIs everywhere. Are you excited to start making calls? check out our API reference for how to make use of Flutterwave APIs.
During your implementation, you can always refer back to this article if there is something you missed. Let us know which other concepts we should cover via Twitter or slack.